Understanding pthread_create: A Key to Multithreading in C
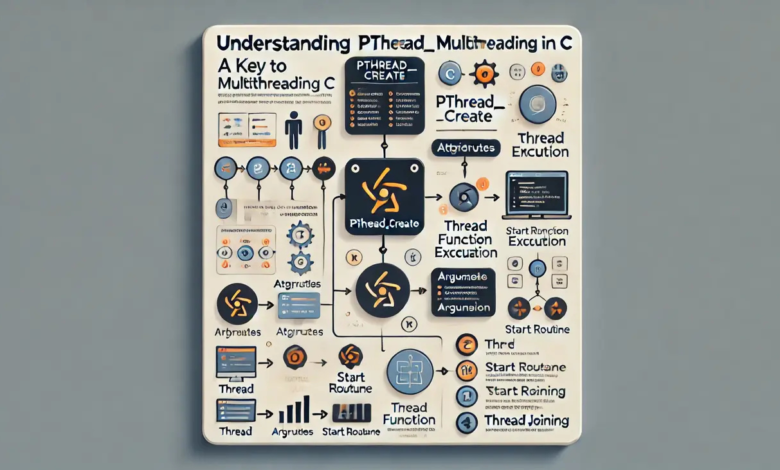
When you dive into multithreading in C, one of the first functions you’ll encounter is pthread_create. This function, part of the POSIX threads (pthreads) library, is crucial for creating new threads within your C programs. In this article, we’ll explore what pthread_create is, how it works, and why it’s essential for multithreaded programming in C.
What is pthread_create?
At its core, pthread_create allows you to create a new thread in a running process. A thread is a unit of execution, and in a multithreaded program, multiple threads can run concurrently, improving performance and responsiveness. The pthread_create function gives you the flexibility to specify which function the new thread should execute, as well as the arguments it needs.
Multithreading can be incredibly useful when you need to perform multiple tasks simultaneously. For instance, a web server can handle multiple requests at the same time by spawning new threads for each request. With pthread_create, you can manage the complexities of thread creation and synchronization.
How Does pthread_create Work?
The process of creating a thread using pthread_create is fairly straightforward, but understanding how to manage the different parameters it accepts is crucial for success.
Function Parameters
- Thread: A pointer to a thread identifier, which stores the ID of the new thread once it’s created.
- Attributes: A pointer to a set of thread attributes. If you don’t need specific attributes, you can pass
NULL
to use the default settings. - Start Routine: This is the function that will be executed by the new thread. It must accept a
void *
argument and return avoid *
result. - Argument: A single argument passed to the start routine. Since C doesn’t allow passing multiple arguments directly to a function, this parameter lets you pass a pointer to any data needed.
When you invoke pthread_create, you are telling the program to spawn a new thread, which will execute the specified function concurrently with the main thread. Once a thread is created, it runs independently, and the main program can continue performing other tasks simultaneously.
Return Value
If pthread_create succeeds, it returns 0
. If it fails, it returns an error code that indicates what went wrong. Understanding and handling these errors properly is key to writing robust multithreaded programs.
Common Pitfalls When Using pthread_create
While pthread_create is a powerful tool, there are some common mistakes that developers make. Let’s go over some of them and how to avoid them.
Passing Loop Variables
A common mistake is passing a loop variable directly as an argument to the thread function. This can lead to unexpected behavior because the loop variable might change its value before the thread starts executing. To avoid this, it’s important to pass a separate variable to each thread.
Thread Synchronization
Multithreading often involves accessing shared resources between threads, which can result in data races if not managed properly. To prevent these issues, synchronization mechanisms like mutexes or semaphores are necessary. Using these tools ensures that only one thread can access a shared resource at a time, preventing corruption of data.
Thread Joining
After creating threads with pthread_create, it’s important to ensure that your main thread waits for them to finish before it terminates. This is done through a process known as “thread joining.” If threads are not joined properly, the main program could exit prematurely, potentially leaving some threads unfinished or resources unfreed.
Why Should You Use pthread_create?
In summary, pthread_create is an essential function for anyone working with multithreading in C. It allows you to create threads, run them concurrently, and improve the efficiency of your programs. Whether you’re building a multithreaded server, a parallel processing application, or a simple program that needs concurrent execution, pthread_create is the tool you’ll rely on.
The ability to spawn multiple threads with pthread_create opens up numerous possibilities for creating high-performance, responsive applications. It’s an indispensable tool for developers looking to make the most of modern, multicore processors, ensuring your programs can handle multiple tasks efficiently at once.
Frequently Asked Questions (FAQs)
What is the difference between a process and a thread in C programming?
A process is an independent program that runs in its own memory space, while a thread is a smaller unit of a process. Threads within the same process share memory, allowing them to communicate with each other more easily. This makes multithreading, like what you can achieve with pthread_create, ideal for tasks that require frequent data sharing and communication.
Can I create multiple threads using pthread_create?
Yes, you can create as many threads as needed by calling pthread_create multiple times, once for each new thread. Each thread created will execute the same function, although you can pass different arguments to each thread if needed.
What happens if pthread_create fails?
If pthread_create fails, it will return a non-zero error code, which can provide information about the failure. Common reasons for failure include lack of system resources or incorrect arguments. You should always check the return value of pthread_create to ensure that the thread is successfully created.
How do I make sure threads don’t interfere with each other?
When using pthread_create, it’s important to manage access to shared resources. You can use synchronization mechanisms like mutexes or condition variables to ensure that only one thread at a time can access a particular resource. This prevents race conditions, where multiple threads try to modify the same data simultaneously.
Do threads created with pthread_create run in parallel?
Yes, if your system has multiple cores, threads created with pthread_create can run in parallel. However, on a single-core processor, the operating system will switch between threads, giving the illusion of parallelism through time-sharing.
Conclusion
In summary, pthread_create is an essential function for anyone working with multithreading in C. It enables you to create new threads within your program, allowing for concurrent execution of multiple tasks. By mastering pthread_create and understanding its parameters, return values, and common pitfalls, you can unlock the full power of multithreading in your applications.
Whether you’re building a complex multithreaded application or just need to handle background tasks more efficiently, pthread_create offers the flexibility and control you need. Remember to handle synchronization carefully and join threads to avoid premature termination of your main program. With these best practices, you’ll be on your way to writing efficient, high-performance multithreaded programs.